scanner(Understanding the Scanner and Its Uses)
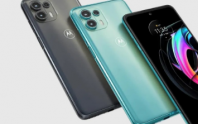
Understanding the Scanner and Its Uses
Introduction:
The scanner is an essential tool for obtaining input from the user in Java programming. It allows the program to read various types of data, such as numbers, strings, and characters, entered by the user during runtime. This article aims to provide a comprehensive understanding of the scanner class, its uses, and how it can be implemented in Java programs.
Overview of the Scanner Class:
The scanner class is part of the java.util package and provides methods for reading input from various sources, including the keyboard. It is a versatile class that can parse primitive types and strings from the input stream. To use the scanner class, we must first import the package and create an instance of the scanner object.
Creating an Instance of the Scanner Object:
To create an instance of the scanner object, we need to import the java.util.Scanner package at the beginning of our program. We can then use the following line of code to create a scanner object:
Scanner scanner = new Scanner(System.in);
The above code creates a scanner object named \"scanner\" that reads input from the system console or keyboard, denoted by \"System.in\". This is the most common usage of the scanner class, but it can also be used to read input from other sources, such as files.
Reading Different Types of Input:
Once we have created an instance of the scanner object, we can use its methods to read different types of input. The scanner class provides various methods for reading different data types, such as nextInt() for integers, nextDouble() for floating-point numbers, and next() for strings.
Example:
Scanner scanner = new Scanner(System.in); System.out.print(\"Enter your age: \"); int age = scanner.nextInt(); System.out.print(\"Enter your name: \"); String name = scanner.next(); System.out.println(\"Hello, \" + name + \"! You are \" + age + \" years old.\");
In the above example, we first prompt the user to enter their age and store it in the \"age\" variable using the nextInt() method. Then we prompt the user to enter their name and store it in the \"name\" variable using the next() method. Finally, we display a personalized greeting using the entered name and age.
Handling Input Mismatch Exceptions:
While using the scanner class, it's important to handle input mismatch exceptions gracefully. If the user enters an input that doesn't match the specified data type, such as entering a string when the program expects an integer, an input mismatch exception will occur. To handle this exception, we can use a try-catch block.
Example:
Scanner scanner = new Scanner(System.in); try { System.out.print(\"Enter your age: \"); int age = scanner.nextInt(); // Rest of the code... } catch (InputMismatchException e) { System.out.println(\"Invalid input! Please enter a valid age.\"); }
In the above example, if the user enters a non-integer value for the age, an InputMismatchException will be thrown. The catch block will then catch this exception and display an appropriate error message to the user.
Common Uses of the Scanner Class:
The scanner class has a wide range of uses in Java programming. Some common uses include:
- Reading user input for interactive programs
- Parsing data from files
- Processing data from network streams
- Scanning tokens in text-based files
Conclusion:
The scanner class is a powerful tool for reading input from various sources in Java programming. It simplifies the process of obtaining user input and provides methods for parsing different types of data. Understanding how to create an instance of the scanner object, reading different types of input, and handling input mismatch exceptions is crucial for effectively using the scanner class in Java programs.
版权声明:本文版权归原作者所有,转载文章仅为传播更多信息之目的,如作者信息标记有误,请第一时间联系我们修改或删除,多谢。