scanner(Scanner A Powerful Tool for Processing User Input)
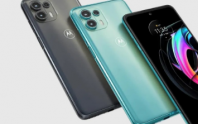
Scanner: A Powerful Tool for Processing User Input
Introduction:
Scanners are an important tool in computer programming for reading user input. They provide a convenient way to read different types of data from a variety of sources, such as the keyboard or a file. This article will explore the capabilities of the Scanner class in Java and explain how it can be used to process user input effectively.
Using the Scanner Class:
Java provides the Scanner class in the java.util package to handle input from various sources. To use the Scanner class, you first need to import it using the import statement:
import java.util.Scanner;
Once imported, you can create an instance of the Scanner class by calling its constructor:
Scanner scanner = new Scanner(System.in);
Here, we are creating a Scanner object that reads input from the standard input stream, which is typically the keyboard. You can also create a Scanner object to read from a file or a string.
Reading Different Data Types:
The Scanner class provides various methods to read different data types. Some of the commonly used methods include:
nextInt()
: Reads an integer value from the input.nextDouble()
: Reads a double value from the input.next()
: Reads a string without spaces from the input.nextLine()
: Reads a complete line of text from the input.
For example, let's say we want to read an integer and a string from the user:
int age = scanner.nextInt();
String name = scanner.next();
These statements will read an integer value into the variable \"age\" and then read a string into the variable \"name\". It is important to note that the next()
method reads only one word, without spaces. If you want to read a sentence or a line of text, you can use the nextLine()
method.
Handling Exceptions:
When using the Scanner class, it is important to handle potential exceptions that can occur during input operations. For example, if the user enters invalid input when an integer is expected, an InputMismatchException will be thrown. To handle such exceptions, you can use the try-catch block:
try {
int num = scanner.nextInt();
// rest of the code
} catch (InputMismatchException e) {
System.out.println(\"Invalid input. Please enter an integer.\");
}
In this example, the code within the try block tries to read an integer, and if an InputMismatchException occurs, it is caught in the catch block and an informative error message is displayed to the user.
Conclusion:
The Scanner class in Java provides a powerful and versatile tool for processing user input. It allows programmers to read different data types from various sources, making it easier to handle user interactions in programs. By properly using the Scanner class and handling exceptions, developers can ensure a smooth and reliable user input experience.
Overall, the Scanner class is an invaluable asset for any programmer working with user input in Java, providing a robust and efficient way to process data.
版权声明:本文版权归原作者所有,转载文章仅为传播更多信息之目的,如作者信息标记有误,请第一时间联系我们修改或删除,多谢。