reversefind(ReverseFind)
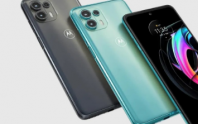
ReverseFind
ReverseFind is a powerful function that is commonly used in programming languages to search for the last occurrence of a specified element or pattern within a given string or sequence. This function is particularly useful when dealing with large datasets or when performance optimization is vital. In this article, we will explore the concept of reverse finding and discuss its applications, advantages, and implementation.
Understanding ReverseFind
ReverseFind is the reverse counterpart of the Find function. While the Find function starts searching from the beginning of a string or sequence for a given element or pattern, the ReverseFind function starts searching from the end, returning the index of the last occurrence found. This function is typically used when we have a need to find the position of the last element in a sequence or string that matches a specific criterion.
The ReverseFind function is available in various programming languages, such as C++, Java, Python, and many others. Its exact syntax and implementation may vary slightly between languages, but the core concept remains the same. By utilizing this function, developers can efficiently search for the last occurrence of an element or pattern, saving valuable time and resources.
Applications of ReverseFind
ReverseFind has a wide range of applications in different domains of programming. Some common applications include:
1. Searching for the last occurrence of a character or substring within a string: ReverseFind allows us to quickly locate the index of the last occurrence of a character or substring within a larger string. This is often helpful when parsing or manipulating textual data.
2. Removing trailing whitespace or other characters: ReverseFind can be used to identify the position of trailing whitespace or other unwanted characters at the end of a string. By knowing the index of the last occurrence of such characters, we can easily remove them from the string.
3. Checking for the presence of a specific element in a sequence: ReverseFind can be used to determine if a specific element exists in a sequence and, if so, at which index. This is helpful in scenarios where we need to validate the presence or absence of certain data.
Advantages of ReverseFind
The use of ReverseFind offers several advantages in terms of efficiency, code readability, and performance optimization. Some key advantages include:
1. Time complexity optimization: By using ReverseFind, we can potentially reduce the search time for the last occurrence of an element or pattern. Since the function starts searching from the end, it can terminate as soon as it finds the first match, eliminating the need to iterate through the entire sequence or string.
2. Improved code readability: ReverseFind provides a clear indication of the programmer's intent to search for the last occurrence of a specific element or pattern. This improves the code's readability and makes it easier for other developers to understand and maintain.
3. Performance optimization: In scenarios where the size of the dataset is enormous, or the search operation is performed frequently, ReverseFind can significantly optimize the overall performance of the program. By avoiding unnecessary iterations and reducing the search space, the program becomes more efficient and responsive.
Implementation of ReverseFind
The implementation of ReverseFind may vary depending on the programming language used. However, the basic approach involves iterating through the string or sequence from the end and comparing each element to the desired element or pattern until a match is found. Once a match is found, the index is returned, or -1 if the element or pattern is not found.
Here is an example implementation of ReverseFind in C++:
int ReverseFind(const std::string& str, char target) {
for (int i = str.length() - 1; i >= 0; i--) {
if (str[i] == target) {
return i;
}
}
return -1;
}
In the above implementation, we start iterating through the string from the end using a for loop. We compare each character to the target character and return the index if a match is found. If no match is found after iterating through the entire string, -1 is returned.
Similar implementations can be created in other programming languages by utilizing the appropriate syntax and looping constructs.
Conclusion
ReverseFind is a valuable function in programming, enabling developers to efficiently search for the last occurrence of an element or pattern within a given string or sequence. Its applications range from simple string manipulation to complex data validation. By understanding and utilizing ReverseFind, developers can enhance code performance, readability, and overall program efficiency.
版权声明:本文版权归原作者所有,转载文章仅为传播更多信息之目的,如作者信息标记有误,请第一时间联系我们修改或删除,多谢。